import tkinter as tk
from tkinter import *
main=tk.Tk()
main.title=("Anleitungen erstellen")
def ohne_aenderung():
print ("ohne Änderung")
def runde():
print ("Runde ")
def reihe():
print ("Reihe ")
def in_den_Maschenring():
print ("Runde 1: 6 fM in den Maschenring")
def zun_mal_6():
print ("Runde 2: Zun * 6")
button1=tk.Button(main, text="o.Ä", command=ohne_aenderung)
button1.pack()
button2=tk.Button(main, text="Runde", command=runde)
button2.pack()
button3=tk.Button(main, text="Reihe", command=reihe)
button3.pack()
button4=tk.Button(main,text="6 fM in Maschring" , command=in_den_Maschenring)
button4.pack()
main.mainloop()
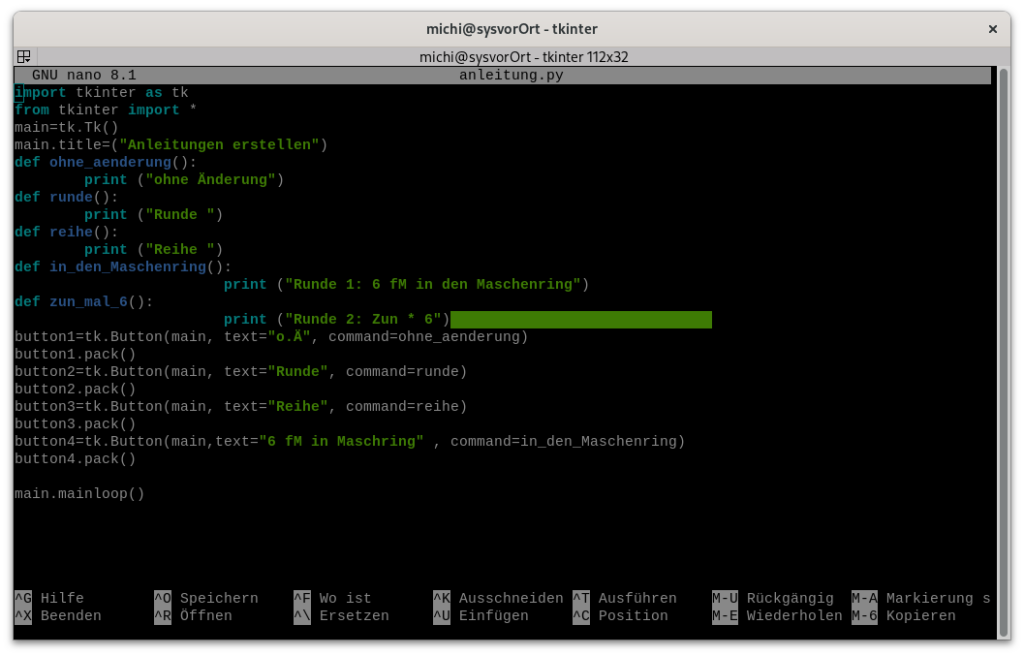
Allerdings wird die Ausgabe dann im Terminal erfolgen. Um dies zu ändern wird der Code geändert und print durch update ersetzt
Mit Tkinter sieht das Design etwas älter aus.
import tkinter as tk
from tkinter import *
# Main-Window
main = tk.Tk()
main.title("Anleitungen erstellen")
# Funktion, um Text in das Textfeld zu schreiben
def update_text(text):
text_widget.insert(END, text )
# Buttons und deren Aktionen
def ohne_aenderung():
update_text("ohne Änderung")
def runde():
update_text("Runde")
def reihe():
update_text("Reihe")
def in_den_Maschenring():
update_text("Runde 1: 6 fM in den Maschenring")
def zun_mal_6():
update_text("Runde 2: Zun * 6 = 12")
def fMZun():
update_text("Runde 3: (1 fM, Zun) * 6 = 18")
def zweifM():
update_text("Runde 4: (2 fM, Zun) * 6 = 24")
def loeschen():
# Alle Zeilen holen
lines = text_widget.get("1.0", END).splitlines()
# Letzte Zeile löschen, wenn's noch was gibt
if lines:
text_widget.delete("end-2l", "end-1l")
# Text-Widget hinzufügen
text_widget = Text(main, height=10, width=50)
text_widget.pack()
# Buttons erstellen
button1 = tk.Button(main, text="o.Ä", command=ohne_aenderung)
button1.pack()
button2 = tk.Button(main, text="Runde", command=runde)
button2.pack()
button3 = tk.Button(main, text="Reihe", command=reihe)
button3.pack()
button4 = tk.Button(main, text="6 fM in Maschring", command=in_den_Maschenring)
button4.pack()
button5 = tk.Button(main, text="Löschen", command=loeschen)
button5.pack()
button6 = tk.Button(main, text="fM,Zun", command=fMZun)
button6.pack()
button7 = tk.Button(main, text="zweifM", command=zweifM)
button7.pack()
main.mainloop()
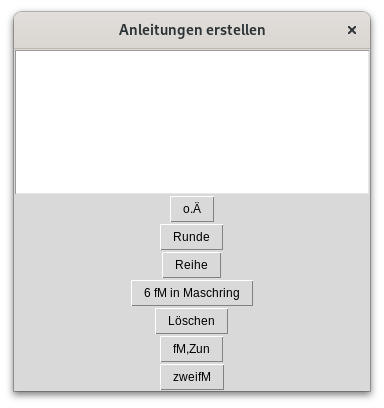
Kleine Anwendung mit Qt:
import sys
from PyQt5.QtWidgets import QApplication, QWidget, QVBoxLayout, QHBoxLayout, QPushButton, QLineEdit, QLabel
from PyQt5.QtGui import QClipboard
class ButtonCreator(QWidget):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
self.setWindowTitle('Button Creator')
self.setGeometry(100, 100, 300, 400)
layout = QVBoxLayout()
# Eingabefelder für Button-Text und zugehörigen Text
self.button_text_input = QLineEdit(self)
self.button_text_input.setPlaceholderText('Button Text')
self.text_input = QLineEdit(self)
self.text_input.setPlaceholderText('Text to copy')
# Button zum Erstellen neuer Buttons
create_button = QPushButton('Create Button', self)
create_button.clicked.connect(self.create_new_button)
layout.addWidget(self.button_text_input)
layout.addWidget(self.text_input)
layout.addWidget(create_button)
# Container für erstellte Buttons
self.button_container = QVBoxLayout()
layout.addLayout(self.button_container)
self.setLayout(layout)
def create_new_button(self):
button_text = self.button_text_input.text()
text_to_copy = self.text_input.text()
if button_text and text_to_copy:
new_button = QPushButton(button_text, self)
new_button.clicked.connect(lambda: self.copy_to_clipboard(text_to_copy))
self.button_container.addWidget(new_button)
# Eingabefelder leeren
self.button_text_input.clear()
self.text_input.clear()
def copy_to_clipboard(self, text):
clipboard = QApplication.clipboard()
clipboard.setText(text)
if __name__ == '__main__':
app = QApplication(sys.argv)
ex = ButtonCreator()
ex.show()
sys.exit(app.exec_())
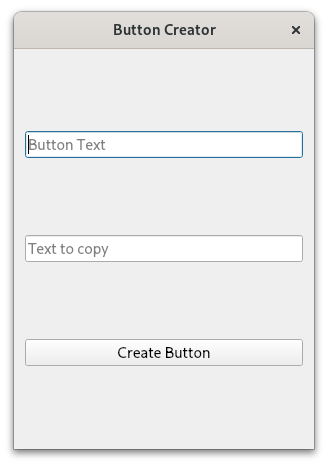