import sys
from PyQt5.QtWidgets import QApplication, QWidget, QVBoxLayout, QHBoxLayout, QPushButton, QLineEdit, QLabel
from PyQt5.QtGui import QClipboard
class ButtonCreator(QWidget):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
self.setWindowTitle('Button Creator')
self.setGeometry(100, 100, 300, 400)
layout = QVBoxLayout()
# Eingabefelder für Button-Text und zugehörigen Text
self.button_text_input = QLineEdit(self)
self.button_text_input.setPlaceholderText('Button Text')
self.text_input = QLineEdit(self)
self.text_input.setPlaceholderText('Text to copy')
# Button zum Erstellen neuer Buttons
create_button = QPushButton('Create Button', self)
create_button.clicked.connect(self.create_new_button)
layout.addWidget(self.button_text_input)
layout.addWidget(self.text_input)
layout.addWidget(create_button)
# Container für erstellte Buttons
self.button_container = QVBoxLayout()
layout.addLayout(self.button_container)
self.setLayout(layout)
def create_new_button(self):
button_text = self.button_text_input.text()
text_to_copy = self.text_input.text()
if button_text and text_to_copy:
new_button = QPushButton(button_text, self)
new_button.clicked.connect(lambda: self.copy_to_clipboard(text_to_copy))
self.button_container.addWidget(new_button)
# Eingabefelder leeren
self.button_text_input.clear()
self.text_input.clear()
def copy_to_clipboard(self, text):
clipboard = QApplication.clipboard()
clipboard.setText(text)
if __name__ == '__main__':
app = QApplication(sys.argv)
ex = ButtonCreator()
ex.show()
sys.exit(app.exec_())
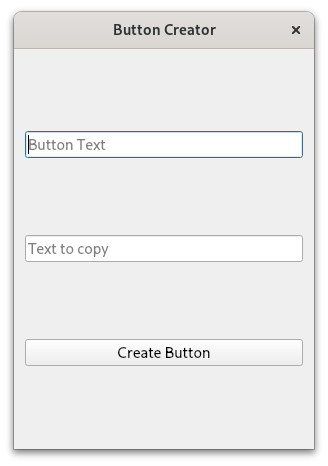